I created an example that renders connections as straight lines: bpmn-js Simple Layout Example - CodeSandbox
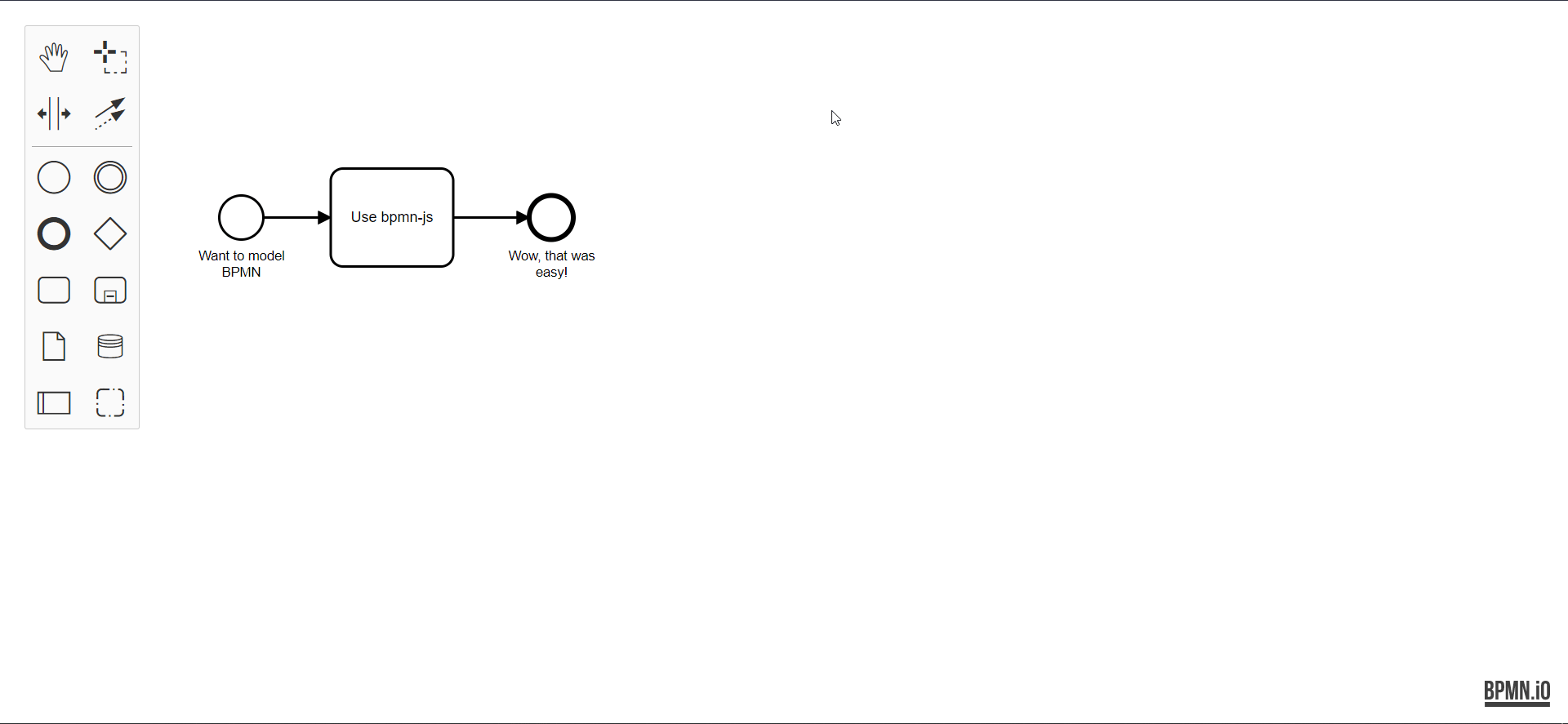
Here’s what I did:
1. Change layout
That one was easy. I simply replaced the layout module with a simpler one that is part of diagram-js.
layouter: ["type", BaseLayouter]
2. Disable Segment Move
Disabling only parts of the bendpoints feature was tricky. We still want to be able to reconnect the start or end of a connection but not move segments or add bendpoints.
First I removed the UI:
.djs-bendpoint.floating,
.djs-segment-dragger {
display: none !important;
}
Then I realized that the segment move
feature cannot be disabled simply by overriding it with an empty module since other modules will try and use this module. So I replaced it with a module that does nothing:
connectionSegmentMove: ["value", { start() {} }],
3. Disable Bendpoint Move
This one was the hardest. The bendpoint move feature is currently implemented in a way that makes it hard to override methods. So I had to use a factory function that creates an instance of this module first and then overrides a method.
function createBendPointMove(
injector,
eventBus,
canvas,
dragging,
rules,
modeling
) {
const bendpointMove = new BendpointMove(
injector,
eventBus,
canvas,
dragging,
rules,
modeling
);
const start = bendpointMove.start.bind(bendpointMove);
bendpointMove.start = (event, connection, bendpointIndex, insert) => {
if (insert) {
return;
}
start(event, connection, bendpointIndex, insert);
};
return bendpointMove;
}
...
bendpointMove: [
"factory",
[
"injector",
"eventBus",
"canvas",
"dragging",
"rules",
"modeling",
createBendPointMove
]
],
This way you can still reconnect the start or end of a connection but not add bendpoints.
Summary
All of your requirements can be implemented due to the flexibility of bpmn-js but sometimes it’s not as straight forward as it should be.